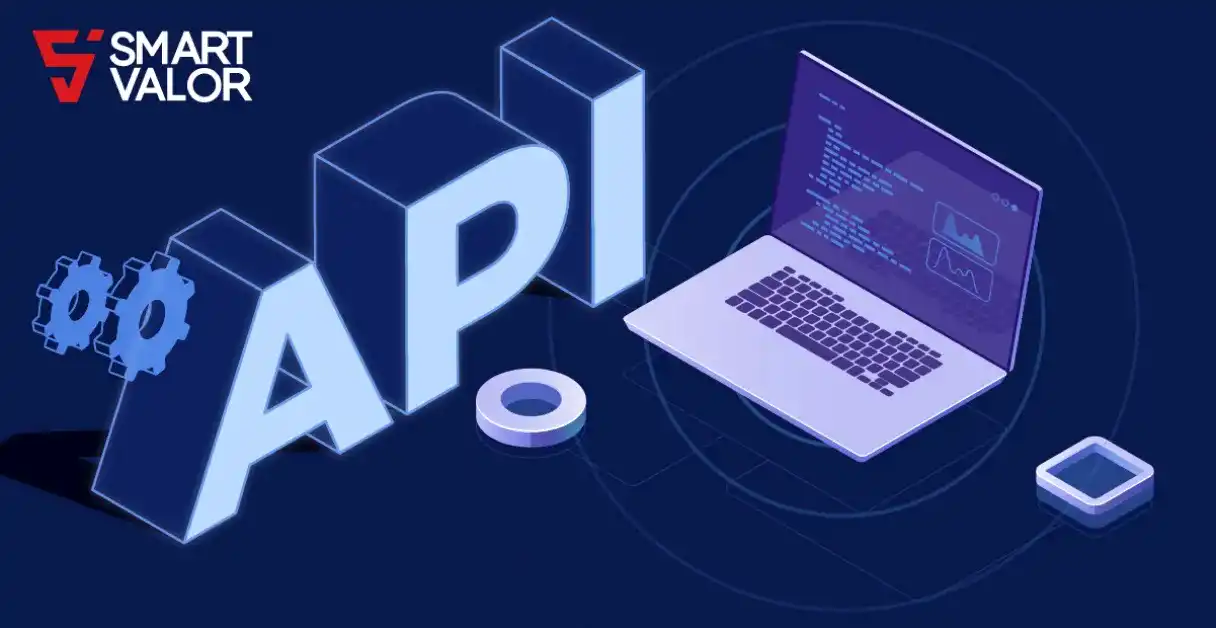
All our customer have free access to our public and easy to use public API. This is the first article of a series to help you to understand the powerful capabilities of API.
How do i get my API Key?
Create through: Settings>API Keys Management>See Available Keys
or for test environment: API Keys
Nodejs:
const crypto = require('crypto');
const apiKey = 'api-key-provided-by-smart-valor';
const secret = 'api-secret-provided-by-smart-valor';
const userId = 99999; // identifier provided by smart valor
const nonce = 1234561; // random number that should change on each call
const signature = crypto
.createHmac('sha256', secret)
.update(`${nonce}${userId}${apiKey}`)
.digest('hex');
console.log('Signature: ', signature);
```
# Use curl or other tool to call the api
## retrieve account balance
curl -X GET "https://api.uat-valor.cc/account/balance" \
-H "accept: application/json" \
-H "api-key: api-key-provided-by-smart-valor" \
-H "nonce: 123456" \
-H "signature: generate-signature-for-the-secret-nonce-and-user-id" \
-H “user-id: 99999”
Full Sample:
const crypto = require('crypto');
const apiUrl = 'https://api.uat-valor.cc';
const apiKey = 'db90737bbe622f5c24485570022a77fe';
const secret = '361dc4db5ea052f223ce38fa5a9a7a13';
const identification = 90; // user_id provided by smart valor
const nonce = parseInt(crypto.randomBytes(4).toString('hex'), 16);
const axios = require('axios');
class Client {
constructor() {
this.signature = crypto.createHmac('sha256', secret).update(`${nonce}${identification}${apiKey}`).digest('hex');
}
getHeaders() {
return {
'Content-Type': 'application/json',
nonce: nonce,
signature: this.signature,
identification: identification,
'api-key': apiKey
};
}
getName() {
return 'SVP Exchange';
}
async getBalance(currency) {
const request = {
url: `${apiUrl}/account/balance`,
method: 'GET',
headers: this.getHeaders()
};
const data = (await this.executeRequest(request)).data;
if (!currency) {
return data;
}
return data.find(c => c.product.isoCode === currency);
}
async getLastPrice(market) {
const instrument = await this.getInstrument(market);
const request = {
url: `${apiUrl}/instruments/${instrument.id}/price`,
method: 'GET',
headers: this.getHeaders()
};
return (await this.executeRequest(request)).data.amount;
}
async getInstrument(market) {
const instruments = await this.getInstruments();
const instrument = instruments.find(i => i.symbol === market);
if (!instrument) {
throw new Error(`No such market found: ${market}`);
}
return instrument;
}
async getInstruments() {
const request = {
url: `${apiUrl}/instruments`,
method: 'GET',
headers: this.getHeaders()
};
return (await this.executeRequest(request)).data;
}
// { id: number, status: string }
async createOrder(market, sellOrBuy, marketOrLimit, price, quantity) {
const request = {
url: `${apiUrl}/orders`,
method: 'POST',
headers: this.getHeaders(),
data: {
side: sellOrBuy,
quantity: quantity,
type: marketOrLimit,
instrumentId: (await this.getInstrument(market)).id,
price: price
}
};
try {
return (await this.executeRequest(request)).data;
} catch (e) {
throw e;
}
}
async getLevel1(market) {
const instrument = await this.getInstrument(market);
const request = {
url: `${apiUrl}/instruments/${instrument.id}/level1`,
method: 'GET',
headers: this.getHeaders()
};
return (await this.executeRequest(request)).data;
}
async getLevel2(market) {
const instrument = await this.getInstrument(market);
const request = {
url: `${apiUrl}/instruments/${instrument.id}/level2`,
method: 'GET',
headers: this.getHeaders()
};
return (await this.executeRequest(request)).data;
}
async getAskPrice(market) {
return (await this.getLevel1(market)).bestOffer;
}
async getBidPrice(market) {
return (await this.getLevel1(market)).bestBid;
}
async isOrderExecuted(orderId) {
const request = {
url: `${apiUrl}/orders/history`,
method: 'GET',
headers: this.getHeaders()
};
const orders = (await this.executeRequest(request)).data;
const order = orders.find(o => o.id === orderId);
if (!order) {
throw new Error(`Order was not found ${orderId}`);
}
return order.status === 'FULLYEXECUTED';
}
async cancelAllOrders() {
const request = {
url: `${apiUrl}/orders`,
method: 'DELETE',
headers: this.getHeaders()
};
return (await this.executeRequest(request)).data;
}
async executeRequest(request) {
const start = new Date();
const response = await axios.request(request);
const end = new Date() - start;
console.info('Execution time [%s] %dms', request.url, end);
return response;
}
}
module.exports = Client;